Use-Cases of this Tutorial
- Javascript library to automatically maintain a proper format for input text while the user is typing (date, time, credits cards etc).
- Javascript library to detect type of credit card based on user input.
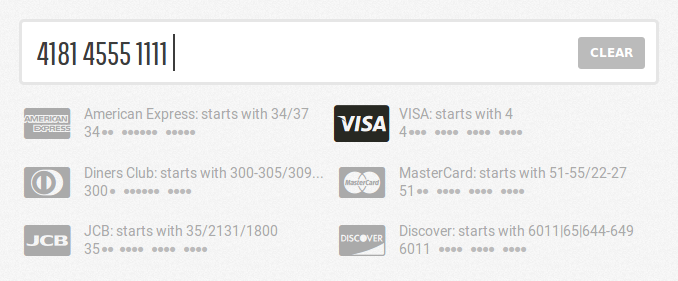
While creating HTML forms, we need to make sure that some types are in a valid format :
- Date & Time : User can enter only specific format like YYYY-MM-DD, hh:mm etc
- Credit Card Numbers : User can enter 16 digits starting with 4 for a Visa card. For an Amex card it will be 15 digits starting with 34/37. etc
- Phone Numbers : User can enter a US phone number of the format 634 634 6346, or of a different format
- Numbers : User can enter a currency as "thousand" style (6,555,555) or "lakh" style (65,55,555)
We can allow the user to enter any input, do a validation and show an error message. But for a better user experience, it would be better if the user can get an idea of the format while he is typing in the text field.
Cleave.js does exactly this — formats an <input type="text" /> while the user is typing.
Demo
Try typing a date of the format YYYY-MM-DD in the below textbox.
You can also find demo for other options.
Types of Options Supported
- Credit Cards : Format as credit card. It can also detect the type of the card - Visa, Master, Amex etc.
- Phone Numbers : Format as phone number of a specific country. It supports formats of most countries.
- Date : Format as date, according to a given pattern (YYYY-MM-DD, YYYY/MM/DD etc)
- Time : Format as time according to a pattern
- Numerals : Format as a numeral. Delimiters can be set for decimals mark, enter a positive number only, set the scale of decimal etc. Lot many options are supported here.
- Custom Formatting : We can also supply our own custom format
Including Cleave.js in Code
The simplest way to use Cleave.js is to directly include its Javascript files. You can include it locally or from a CDN such as cdnjs.
You can also install it as a npm module. Components are also available for ReactJS and Angular.
Code for using Cleave.js would look something like :
// format as 'YYYY-MM-DD'
var yyyymmdd = new Cleave('#cleave-text', {
date: true,
delimiter: '-',
datePattern: ['Y', 'm', 'd']
});
More Information
The complete documentation, options and demos for Cleave.js can be found here.